- Published at
Mario Python Cheatsheet
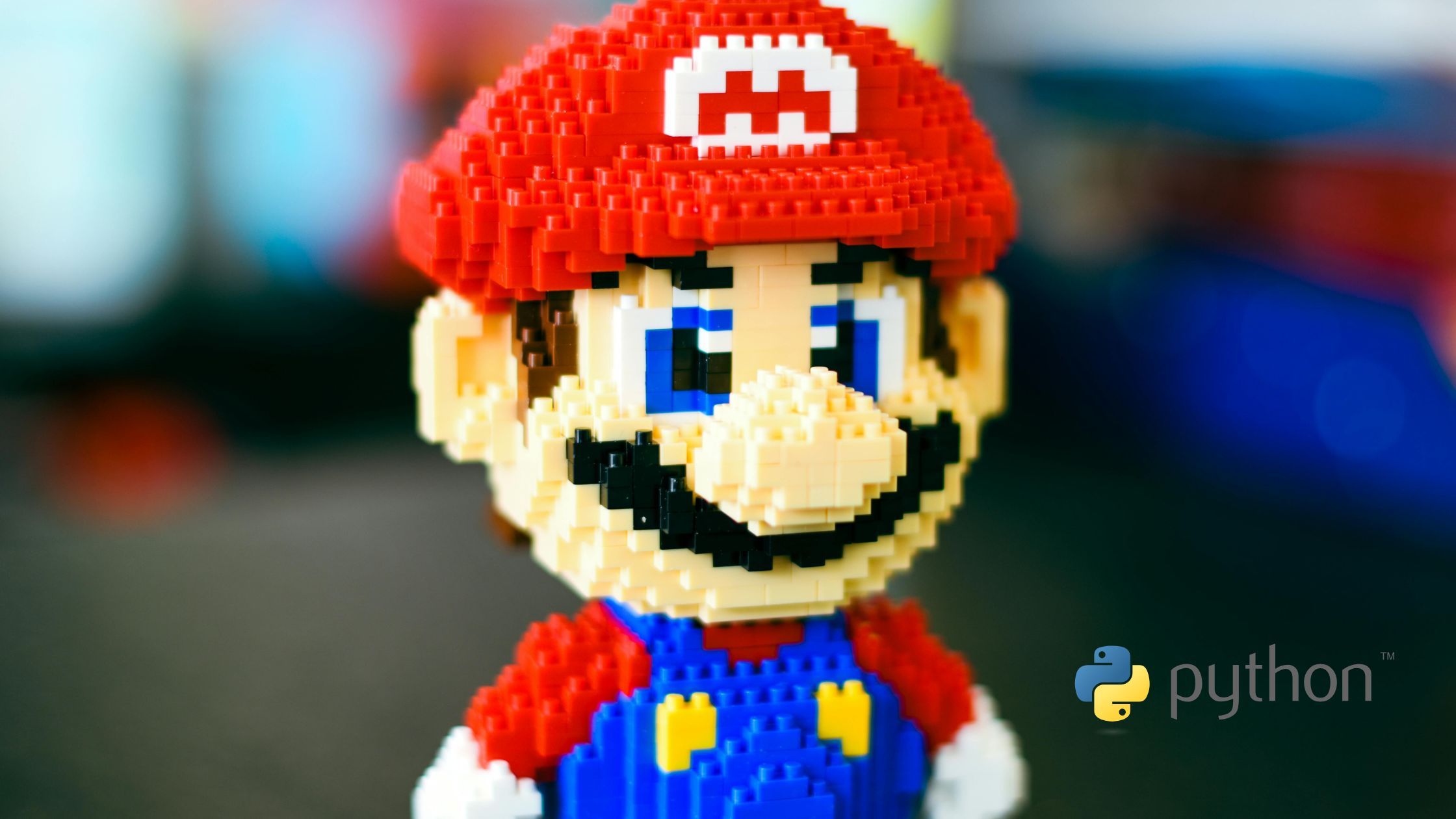
The Python cheatsheet I wish I had when I first started learning Python.
Table of Contents
Introduction
When I first started learning Python, I found myself constantly Googling the basics. I’d make a little progress here and there, but found myself stuff stuck on simple, quick items. As a huge Nintendo and Mario fan, I created this fun cheatsheet that explains Python concepts using examples from Mario Bros.
Python Cheatsheet
Data Types
# Numberscoins = 42 # integerjump_height = 3.14 # floatstar_power = 1 + 2j # complex number
# Stringscharacter = "Mario" # can use single or double quotesmario_quote = """It's-a me,Mario!"""
# Booleanshas_mushroom = Trueis_small = False
# None typeempty_block = None # represents absence of value
Variables
# Basic assignmentlives = 3player_name = "Luigi"
# Multiple assignmentcoins, stars, mushrooms = 100, 5, 2
# Variable naming rules:mario_bros = "OK" # Can use letters, numbers, underscores_princess = "OK" # Can start with underscore1up = "NOT VALID" # Cannot start with number
Operators
# Arithmeticcoins + bonus_coins # Additionlives - damage # Subtractioncoins * multiplier # Multiplicationpoints / divisor # Divisioncoins // bowser # Floor divisionscore % high_score # Modulus (remainder)mushroom ** power_up # Exponentiation
# Comparisonmario == luigi # Equal tobowser != peach # Not equal toscore > high_score # Greater thancoins < target # Less thanpoints >= threshold # Greater than or equal tolives <= max_lives # Less than or equal to
# Logicalhas_star and has_flower # True if both are truehas_mushroom or is_fire # True if at least one is truenot is_small # Inverts boolean value
String Operations
# String concatenationcharacter = "Mario"action = "jumps"message = character + " " + action # "Mario jumps"
# String methodsname = "bowser"name.upper() # "BOWSER"name.capitalize() # "Bowser"name.replace('b', 'w') # "wowser"
# String formattingcharacter = "Mario"coins = 99# f-strings (Python 3.6+)print(f"{character} collected {coins} coins!")
# String slicingworld = "World 1-1"world[0] # 'W'world[0:5] # 'World'world[-3:] # '1-1'
Basic List Operations
# Creating listscharacters = ["Mario", "Luigi", "Peach", "Yoshi"]inventory = ["Mushroom", 3, "Star", True]
# List operationscharacters.append("Toad") # Add to endcharacters.pop() # Remove and return last itemcharacters.insert(0, "Wario") # Insert at specific positionlen(characters) # Get length of list
# List slicingmario_bros = characters[0:2] # Get first two elementsreversed_chars = characters[::-1] # Reverse the list
Tuples
# Creating tuples (immutable lists)coordinates = (3, 5) # Mario's position in levelpipe_location = (100, 200, "Underground")single_item = (1,) # Need comma for single-item tuple
# Accessing tuple elementsx, y = coordinates # Tuple unpackingworld_coords = pipe_location[:2] # First two elements
# Common tuple operationslen(pipe_location) # Number of elements"Underground" in pipe_location # Check if value existsmario_pos = coordinates + (0,) # Combine tuples
Dictionaries
# Creating dictionaries
character_stats = { "Mario": {"lives": 3, "coins": 0, "power": "Fire"}, "Luigi": {"lives": 3, "coins": 0, "power": "None"}}
power_ups = { "Mushroom": "Super Mario", "Fire Flower": "Fire Mario", "Star": "Invincible"}
# Accessing and modifyingmario_lives = character_stats["Mario"]["lives"]power_ups["Ice Flower"] = "Ice Mario" # Add new itemdel power_ups["Mushroom"] # Remove item
# Dictionary methodsall_powers = power_ups.keys()power_effects = power_ups.values()power_items = power_ups.items() # Get key-value pairs
# Check if key existsif "Star" in power_ups: print("Star power available!")
Sets
# Creating sets (unique items only)available_characters = {"Mario", "Luigi", "Peach", "Yoshi"}unlocked_worlds = {1, 2, 3}
# Set operationsavailable_characters.add("Toad")available_characters.remove("Peach")
# Set mathematicsplayer1_items = {"Mushroom", "Star", "Coin"}player2_items = {"Flower", "Mushroom", "Shell"}
both_have = player1_items & player2_items # Intersectionall_items = player1_items | player2_items # Unionunique_to_player1 = player1_items - player2_items # Difference
Basic Functions
# Defining a functiondef power_up(character): return f"{character} got a Super Star!"
# Function with default parameterdef collect_coins(amount=100): return f"Collected {amount} coins!"
# Function with multiple parametersdef calculate_score(coins, time_left): return coins * time_left
# Calling functionspower_up("Mario") # "Mario got a Super Star!"collect_coins() # "Collected 100 coins!"calculate_score(50, 120) # 6000
For Loops
# Basic for loopfor character in ["Mario", "Luigi", "Peach"]: print(f"{character} is ready to play!")
# Range-based for loopfor world in range(1, 9): print(f"Starting World {world}")
# Looping through dictionariesfor character, stats in character_stats.items(): print(f"{character} has {stats['lives']} lives")
# Enumerate for index and valuefor level, boss in enumerate(["Bowser Jr", "Kamek", "Bowser"], 1): print(f"Level {level} Boss: {boss}")
While Loops
# Basic while looplives = 3while lives > 0: print(f"Lives remaining: {lives}") lives -= 1
# Break and continuecoins = 0while True: coins += 1 if coins == 50: print("Got a 1-Up!") continue if coins >= 100: print("Extra life earned!") break
# Game-style loopplayer_power = "Small"while player_power != "Super": print("Looking for mushroom...") found_item = "Mushroom" # Simulated item find if found_item == "Mushroom": player_power = "Super" print("Power up!")
Putting It All together
Here’s an example to tie everything together.
# Track Mario's progress through a leveldef play_level(level_number): player = { "character": "Mario", "power": "Small", "coins": 0, "position": 0 }
obstacles = [ ("Goomba", 50), ("Pipe", 100), ("Koopa", 150), ("Flag", 200) ]
for obstacle, position in obstacles: player["position"] = position
if obstacle == "Flag": print("Level Complete!") break
print(f"Encountered {obstacle} at position {position}")
if player["power"] == "Small": print("Better find a mushroom!")
# Level progression systemworlds = { 1: {"levels": 4, "unlocked": True}, 2: {"levels": 4, "unlocked": False}, 3: {"levels": 4, "unlocked": False}}
for world_num, world_info in worlds.items(): if not world_info["unlocked"]: continue
print(f"World {world_num}") for level in range(1, world_info["levels"] + 1): print(f"Playing level {world_num}-{level}")
Final Thoughts
-
Use meaningful variable names - player_lives is better than pl
-
Use comments to explain WHY, not WHAT (the code should be self-explanatory)
-
Start with simple built-in functions before moving to complex libraries
-
Practice using the interactive Python shell (REPL) to test small code snippets
Remember, everyone begins with the basics. I’m a big believer in learning the fundamentals before branching out to more advanced topics. Coding has never been my strongest skill so practice and persistence are key. More to come as I have some fun project ideas in mind for 2025!